Prepare your platform
The UNIPaaS components are a set of embedded UI components that you can use within your platform. The UNIPaaS components include an out-of-the-box UI and logic. Implementing these within your platform will provide a tremendous amount of value to your customers, as well as dramatically reduce the amount of time and effort required for the implementation of a full payment system.
The following embedded components are available for your platform:
Component name | Description | Priority |
---|---|---|
Pay Portal | Your users' main payments page. It includes a transactions list, current balance, configurations and more. | Required |
Notification | Provides your users with all relevant payment-related notifications, within a user-friendly notification center. | Required Alternatively, if you have your own a notification center, use the notification/create webhook to receive payments notifications |
Invoice | Added to your invoice creation, editing and viewing pages. It provides vital information and configurations regarding the available payment methods for the specific invoice. | Highly recommended |
Balance | Presents your users with the most important payments and registration information on their dashboard. | Highly recommended |
Onboarding | Provides a ready UI for your platform that handles a full vendor registration process, including all KYB/KYC aspects | Required |
Preparing your platform for UNIPaaS components integration
This guide includes the information and steps you need to take to prepare your platform for the UNIPaaS components integration.
Completing the following steps will create the required infrastructure to pass configurations and render all the UNIPaaS components that will be embedded in later stages, manage your access token and listen to events.
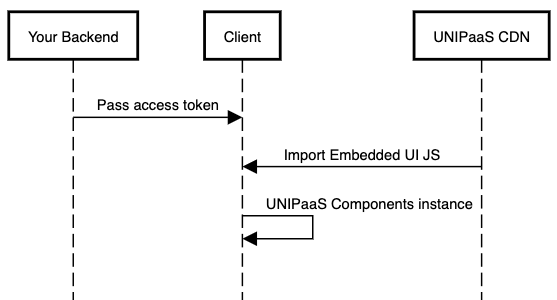
UNIPaaS Components sequence diagram
UNIPaaS access token
The UNIPaaS components access token is required to load any embedded components within your platform. The access token is generated in your backend by making an authorize API call, with defined scopes and a vendor ID (if you have a vendor ID).
Scopes define the permissions your access token will grant for all of the UNIPaaS components you will be using throughout your platform. When creating your access token, consider which of the UNIPaaS components you will be using in your platform.
The following scopes are available:
Scopes that cover all UNIPaaS Components permissions
Use the following scopes to allow your users access to all UNIPaaS Components on your platform.
Scope name | Description |
---|---|
portal_read | All GET permissions required for using UNIPaaS Components on your platform |
portal_write | All POST permissions required for using UNIPaaS Components on your platform |
Specific component scopes
In case there is a need to grant permissions to specific component only, use the following granular scopes to customize permissions according to your needs:
Scope name | Description |
---|---|
onboarding_read | Embedded onboarding component GET permissions |
onboarding_write | Embedded onboarding component POST permissions |
ewallet_read | Embedded Balance component GET permissions |
payout_write | Embedded Balance component payout features POST permissions |
payout_read | Embedded Balance component payout features GET permissions |
link_read | Embedded Balance component additional features GET permissions |
link_write | Embedded Balance component additional features POST permissions |
notification_read | Embedded Notification component additional features GET permissions |
notification_write | Embedded Notification component additional features POST permissions |
invoice_read | Embedded Invoice component additional features GET permissions |
invoice_write | Embedded Invoice component additional features POST permissions |
UNIPaaS components DOM events
UNIPaaS components will communicate with your platform by firing front end events. Use these events to link your users to different pages on your platform, handle exceptions, errors and more.
Event name | Description | Your action | Event action type |
---|---|---|---|
startOnboarding | A new user's intent to register to your payments platform (new users only) | Redirect to platform’s create new vendor flow | Link |
completeOnboarding | User’s intent to complete registration with UNIPaaS | Redirect to the UNIPaaS embedded onboarding component on your platform | Link |
invoicePage Payload: {invoiceId: String} Example: { invoiceId: "INV1234" } | User’s intent to view a specific invoice. This event includes an invoice number as payload | Redirect to the specific invoice page on your platform | Link |
buyerPage Payload: {buyerId: String} Example: { buyerId: "B12345" } | User’s intent to view a specific buyer's profile. This event includes a buyer id as payload | Redirect to the specific buyer (customer) page on your platform | Link |
payPortal Payload: {queryParam?: String} Example: { queryParam: "page=notifications" } | User’s intent to view the Pay Portal (embedded component) or a specific tab of it | Redirect to the UNIPaaS embedded Pay Portal on your platform Add a payload content as a query param to Pay Portal page URL's query string | Link |
enablePayments | User's intent to enable the UNIPaaS-powered payments solution in your platform (while it is disabled) | Redirect to the settings page on your platform, where users can enable (and set as default) the payment solution | Link |
generalError | An exception or error has occurred on the backed during a request | Refresh the page (optional) | Error |
sessionExpired | The access token has expired or invalid access token scope | Refresh the page (optional) | Error |
Expiration
Each access token is valid for 1 hour. Access tokens will auto refresh every time a component communicates with the UNIPaaS servers
Integration steps
Generate the UNIPaaS components access token from your backend.
curl -X POST "https://sandbox.unipaas.com/platform/authorize"
-H "accept: application/json"
-H "Authorization: Bearer <PLATFORM_SECRET_KEY>"
-H "Content-Type: application/json"
--data-raw '{
"scopes": ["onboarding_write", "onboarding_read"], // Replace with relevant scopes
"vendorId": "{vendorId}" // When no vendor ID is sent, you will receive a public access token
}'
The following is an access token request response example:
{
"accessToken": "{{accessToken}}",
"expiresIn": 3600,
"vendorId": "{{vendorId}}",
"merchantId": "{{merchantId}}",
"env": "sandbox",
"scopes": [
"onboarding_read",
"onboarding_write"
]
}
Implement the UNIPaaS components JS in your web pages
Place the following script tag element inside of the <head>
of your HTML page:
<script type="application/javascript" src="https://cdn.unipaas.com/embedded-ui.js"></script>
Place the following script tag element before the </body>
of your HTML page:
-
It's important to pass a boolean value for the
paymentsEnabled
field according to the vendor's default payment provider configuration (True - the default payment provider is UNIPaaS, False - other) -
Customize the appearance of your components using the
theme
fields, this will affect the look of all your embedded components
<script type="text/javascript">
const config = {
paymentsEnabled: true, // mandatory: is UNIPaaS set as a default payment provider
theme: { //optional
colors: { //optional
primaryColor: "#2F80ED", //optional
secondaryColor: "#687B97", //optional
accentTextColor: "#2F80ED", //optional
primaryButtonColor: "#2F80ED" //optional
},
boxShadow: "0px 3px 15px rgba(27, 79, 162, 0.11)" //optional
}
}
const components = unipaas.components("<accessToken>", config);
</script>
Listen (and take action) to UNIPaaS components DOM events as per the following example:
components.on("payPortal", (e) => {
console.log("payPortal event", e.detail);
});
Updated over 2 years ago
Your platform is now ready to implement the first embedded component.
We highly recommend implementing the Pay Portal first.
This core component provides your users with the most critical information about their payments, such as a transactions list, current balance, configurations and more.