Vendor UI: Implementation Guide
UI Web-Embeds offer an efficient and user-friendly way to integrate various functionalities into a web application, without requiring extensive coding effort. By embedding pre-built components directly into your platform's UI, you can save development time and resources, while also ensuring a consistent user experience across your platform thanks to customisation options.
When starting the integration process, it is important to select the components that you intend to implement. This will allow you to handle DOM Events correctly, establish clear boundaries from the outset and successfully complete the integration.
Steps
- Authorization
- Installation
- Implementation of UI Web-Embeds
- Updating UI Web-Embeds
- DOM EventListeners
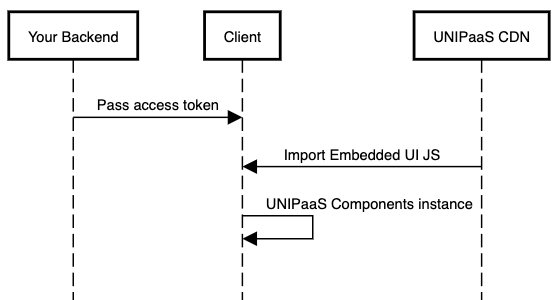
1. Authorization
In order to load UI Web-Embeds in your platform, an authorized API call is needed in your backend with defined scopes and a vendor ID (if applicable) to generate the UNIPaaS access token.
Generate UI components access token
`curl -X POST "https://sandbox.unipaas.com/platform/authorize"
2 -H "accept: application/json"
3 -H "Authorization: Bearer <PLATFORM_SECRET_KEY>"
4 -H "Content-Type: application/json"
5 --data-raw '{
6 "scopes": ["portal_read","portal_write","onboarding_write","invoice_read","invoice_write"],
7 "vendorId": "{vendorId}"
8 }'`
const request = require("request");
const url = "https://sandbox.unipaas.com/platform/authorize";
const secretKey = "<PLATFORM_SECRET_KEY>";
const vendorId = "<YOUR_VENDOR_ID>";
const scopes = ["portal_read", "portal_write", "onboarding_write" , "invoice_read" , "invoice_write"]
const options = {
url,
method: "POST",
headers: {
"Accept": "application/json",
"Authorization": `Bearer ${secretKey}`,
"Content-Type": "application/json"
},
body: JSON.stringify({
scopes,
vendorId
})
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
Verb | POST |
Environment | sandbox |
URL | https://sandbox.unipaas.com/platform/authorize |
Name | Type | Required | Description |
---|---|---|---|
scopes | string | YES | The scopes portal_read , portal_write , onboarding_write , invoice_read and invoice_write included in this API request example, determine the level of access required to perform specific actions, similar to GET and POST methods |
vendorReference | string | YES | The user id on your system |
vendorId | string | NO | A vendor ID is a unique identifier for the vendor requesting API access. You can obtain a vendor ID when you create a vendor on your platform Note! If you don't have a vendor ID, do not include the parameter in your API request, then a public access token will be issued |
Note!
If you don't have a vendor ID, do not include the parameter in your API request, then a public access token will be issued.
Response example
{
"accessToken": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzY29wZXMiOlsib25ib2FyZGluZ193cml0ZSIsInBheW91dF93cml0ZSIsImxpbmtfd3JpdGUiLCJvbmJvYXJkaW5nX3dyaXRlIiwibm90aWZpY2F0aW9uX3dyaXRlIiwiY29uZmlnX3JlYWQiLCJkaXJlY3RfZGViaXRfd3JpdGUiLCJld2FsbGV0X3JlYWQiLCJwdWJsaWNfY29uZmlnX3JlYWQiLCJsaW5rX3JlYWQiLCJvbmJvYXJkaW5nX3JlYWQiLCJub3RpZmljYXRpb25fcmVhZCIsImNvbmZpZ19yZWFkIl0sInZlbmRvcklkIjoiNjNkN2E2Njk4NGFkZDc5YWU2YmFmZDg3IiwibWVyY2hhbnRJZCI6IjYxZTZlOTdmZjEyNDJiODIwYzlkNWM4MSIsImVudiI6ImRldiIsImlhdCI6MTY4MTg1MTQ5MCwiZXhwIjoxNjgxODUzMjkwfQ.q5dCR3zfuEWCTG8HdvfEtlY7BmHxvJL0PFinlJSe8uQ",
"expiresIn": 3600,
"scopes": [
"portal_read",
"portal_write",
"onboarding_write"
],
"vendorId": "63d7a66984add79ae6bafd87",
"merchantId": "61e6e97ff1242b820c9d5c81",
"env": "sandbox"
}
Name | Type | Description |
---|---|---|
accessToken | string | Access token for the UI Web-Embeds |
expiresIn | string | Time until the access token expires (in seconds) |
scopes | string | List of permissions granted for the access token |
vendorId | string | Unique identifier for the vendor requesting access |
merchantId | string | Unique identifier for the platform associated with the vendor |
env | string | The environment in which the access token was issued |
This access token is valid for one hour, and it will be automatically refreshed whenever a UI Web-Embed communicates with the UNIPaaS servers.
Scopes
Name | Description |
---|---|
portal_read | Grants all GET permissions required for using UI Web-Embeds on your platform |
portal_write | Grants all POST permissions required for using UI Web-Embeds on your platform |
onboarding_write | Grants specific POST permissions required for using Onboarding UI Web-Embeds on your platform |
2. Installation
Script tag
Start with placing the following script tag element inside of the <head>
of your HTML page:
<script type="application/javascript" src="https://cdn.unipaas.com/embedded-ui.js"></script>
This script tag loads the JavaScript code that provides the functionality for implementing UNIPaaS UI Web-Embeds on a webpage. When the script is loaded and executed, it will create an object in the memory that contains methods for instantiating and interacting with the UI Web-Embeds.
General configuration
Place the following script tag element below the closing </body>
tag of your HTML page.
This script is used to initialize and configure UNIPaaS UI Web-Embeds on a web page.
<script type="text/javascript">
const config_general = {
paymentsEnabled: true,
theme: {
colors: {
primaryColor: "#2F80ED",
secondaryColor: "#687B97",
accentTextColor: "#2F80ED",
primaryButtonColor: "#2F80ED"
},
fontFamily: "inherit",
boxShadow: "0px 3px 15px rgba(27, 79, 162, 0.11)"
}
}
const components = unipaas.components("<accessToken>", config_general);
</script>
Name | Type | Required | Description |
---|---|---|---|
paymentsEnabled | boolean | YES | Indicates whether the UNIPaaS-powered embedded payments are enabled as the default payment provider for the vendor associated with the API request If set to true , it means that the UNIPaaS-powered embedded payments are available for useIf set to false , it means that the vendor has chosen another payment provider/no online payments as a default option |
Theme
Theme customisation allows to match the visual design of the components with your product.
Name | Type | Description |
---|---|---|
colors.primaryColor | string | Represents the primary color used for UI Web-Embeds |
colors.secondaryColor | string | Represents the secondary color used for UI Web-Embeds |
colors.accentTextColor | string | Represents the font color used for headers of UI Web-Embeds |
colors.primaryButtonColor | string | Represents the font color used for primary buttons of UI Web-Embeds |
fontFamily | string | Represents the font family used for UI Web-Embeds Please use inherit to align the font family to the one used in your platform |
boxShadow | string | Represents the box shadow applied to UI Web-Embeds, used to create a shadow effect |
3. Implementation of UI Web-Embeds
To gain more knowledge of the UI Web-Embeds logic and identify the appropriate location for implementing them within your platform, please refer to the UI Web-Embeds overview.
Note!
Please note that when mounting a component using a selector, the component should not be mounted more than once per selector. Mounting a component more than once can lead to unexpected behavior and issues.
To avoid this, make sure to check whether the component has already been mounted before calling the mount method.
Onboarding
Allocate the minimal space for this component as follows:
Width - 450 pixels
Create a container
Place the following script tag element below the closing </body>
tag of your HTML page.
Make sure to assign a unique ID to the container so that it can be easily identified and accessed.
<div id="onboarding"></div>
Create and mount an instance below the container
Create an instance of it and mount it to the container DOM node in your page. This should be done after the previous div has finished loading.
const onboarding = components.create("onboarding");
onboarding.mount("#onboarding");
Pay portal
Allocate the minimal space for this component as follows:
Width - 970 pixels
Height - 900 pixels
Create a container
Place the following div tag element inside the <body>
tag of your HTML page.
Make sure to assign a unique ID to the container so that it can be easily identified and accessed.
<div id="pay_portal"></div>
Create and mount an instance below the container
Place the following script tag element below the closing </body>
tag of your HTML page.
Create an instance of it and mount it to the container DOM node in your page. This should be done after container (the previous div) has finished loading.
<script type="text/javascript">
const payPortal = components.create("payPortal");
payPortal.mount("#pay_portal");
</script>
Umount an instance
To unmount the instance please use the following method:
<script type="text/javascript">
payPortal.unmount();
</script>
View code sample available on UNIPaaS Github
Notification
Allocate the minimal space for this component as follows (for the clickable icon):
Width - 30 pixels
Height - 30 pixels
Create a container
Place the following div tag element inside the <body>
tag of your HTML page.
Make sure to assign a unique ID to the container so that it can be easily identified and accessed.
<div id="notification"></div>
Create and mount an instance below the container
Place the following script tag element below the closing </body>
tag of your HTML page.
Create an instance of it and mount it to the container DOM node in your page. This should be done after container (the previous div) has finished loading.
<script type="text/javascript">
const config_notif = {
icon: {
url: 'https://cdn.unipaas.com/img/bell-icon.svg',
size: 30
}
};
const notification = components.create("notification", config_notif);
notification.mount("#notification");
</script>
Name | Type | Required | Description |
---|---|---|---|
icon.url | string | NO | Bell icon URL |
icon.size | string | NO | Bell icon height and width in pixels |
Invoice
Invoice UI Web-Embed should be implemented within 3 types of pages:
- New sales invoice - typically includes fields for inputting customer information, item descriptions, prices, and other relevant details related to the sales invoice creation process.
- Edit sales invoice - typically includes fields for modifying an existing sales invoice information, item descriptions, prices, and other relevant details related to the sales invoice.
- View sales invoice - typically includes fields for viewing an existing sales invoice information.
Note!
Allocate the minimal space for this component as follows:
Width - 475 pixels
Height - 250 pixels
New sales invoice page
Create a container
Place the following div tag element inside the <body>
tag of your HTML page.
Make sure to assign a unique ID to the container so that it can be easily identified and accessed.
<div id="invoice"></div>
Create and mount an instance below the container
Place the following script tag element below the closing </body>
tag of your HTML page.
Create an instance of it and mount it to the container DOM node in your page. This should be done after container (the previous div) has finished loading.
<script type="text/javascript">
const config_invoice = {
mode: 'create',
customer: {
reference: '1234567',
name: 'John Doe',
email: '[email protected]',
},
invoice: {
reference: 'INV-123',
}
}; // UNIPaaS Components Invoice config type
const invoice = components.create("invoice", config_invoice);
invoice.mount("#invoice")
</script>
Name | Type | Required | Description |
---|---|---|---|
mode | string | YES | Indicates the type of the invoice page - is the user creating a new invoice, editing or viewing an existing invoice For creating a new sales invoice mode 'create' is required |
customer.reference | string | YES* | Represents the unique identifier for the customer associated with the invoice. * Required for Direct Debit. |
customer.name | string | YES* | Represents the name of the customer associated with the invoice. * Required for Direct Debit. |
customer.email | string | NO | Represents the email address of the customer associated with the invoice. |
invoice.reference | string | YES | Represents the unique identifier for the invoice. |
Update invoice method
Edit & View Sales Invoice
Create a container
Place the following div tag element inside the <body>
tag of your HTML page.
Make sure to assign a unique ID to the container so that it can be easily identified and accessed.
<div id="invoice"></div>
Create and mount an instance below the container
Place the following script tag element below the closing </body>
tag of your HTML page.
Create an instance of it and mount it to the container DOM node in your page. This should be done after container (the previous div) has finished loading.
<script type="text/javascript">
const config_invoice = {
mode: 'edit',
customer: {
reference: '1234567',
name: 'John Doe',
email: '[email protected]',
},
invoice: {
reference: 'INV-123',
}
};
const invoice = components.create("invoice", config_invoice);
invoice.mount("#invoice");
</script>
Name | Type | Required | Description |
---|---|---|---|
mode | string | YES | Indicates the type of the invoice page. Is the user is creating a new invoice, editing or viewing an existing invoice. For editing an existing invoice mode: 'edit' is required.For viewing an existing invoice mode: 'view' is required. |
customer.reference | string | YES* | Represents the unique identifier for the customer associated with the invoice. * Required for Direct Debit. |
customer.name | string | YES* | Represents the name of the customer associated with the invoice. * Required for Direct Debit. |
customer.email | string | NO | Represents the email address of the customer associated with the invoice. |
invoice.reference | string | YES | Represents the unique identifier for the invoice. |
Update invoice method
Update invoice method
When a customer is selected during invoice creation or editing, it is important to use the invoice.update
method. This will let UNIPaaS know which payment options are available for the selected customer, and will change the invoice component in real time to reflect this.
It should be used while users are filling out the mandatory invoice fields using your platform's UI, before they save a new invoice.
Please call the update method with the customer data (you can call this method multiple times with the customer object or with the invoice object separately).
invoice.update({
customer: {
reference: '1234567',
name: 'John Doe',
email: '[email protected]',
},
invoice: {
reference: 'INV-123',
}
})
Name | Type | Required | Description |
---|---|---|---|
customer.reference | string | YES* | Represents the unique identifier for the customer associated with the invoice. * Required for Direct Debit. |
customer.name | string | YES* | Represents the name of the customer associated with the invoice. * Required for Direct Debit. |
customer.email | string | NO | Represents the email address of the customer associated with the invoice. |
invoice.reference | string | NO | Represents the unique identifier for the invoice. |
Important!
Whenever there is a customer selected, you should update the invoice. This applies to when a customer is changed (customer A was selected and now customer B is selected) as well as if there is a default customer selection (customer C is selected by default when the invoice page loads).
Balance
Allocate the minimal space for this component as follows:
Width - 490 pixels
Height - 420 pixels
Create a container
Place the following div tag element inside the <body>
tag of your HTML page.
Make sure to assign a unique ID to the container so that it can be easily identified and accessed.
<div id="balance"></div>
Create and mount an instance below the container
Place the following script tag element below the closing </body>
tag of your HTML page.
Create an instance of it and mount it to the container DOM node in your page. This should be done after container (the previous div) has finished loading.
<script type="text/javascript">
const balance = components.create("balance");
balance.mount("#balance");
</script>
Mandate request
Allocate the minimal space for this component as follows:
Width - 520 pixels
Height - 665 pixels
Create a container
Place the following div tag element inside the <body>
tag of your HTML page.
Make sure to assign a unique ID to the container so that it can be easily identified and accessed.
<div id="request_mandate"></div>
Create and mount an instance below the container
Place the following script tag element below the closing </body>
tag of your HTML page.
Create an instance of it and mount it to the container DOM node in your page. This should be done after container (the previous div) has finished loading.
<script type="text/javascript">
const config_mandate = {
customer: {
reference: '1234567',
name: 'John Doe',
email: '[email protected]',
}
};
const request_mandate = components.create("requestMandate", config_mandate);
request_mandate.mount("#request_mandate");
</script>
Name | Type | Required | Descripton |
---|---|---|---|
customer.reference | string | YES | Represents the unique identifier for the customer on your platform associated with the mandate request. |
customer.name | string | YES | Represents the name of the customer associated with the mandate request. |
customer.email | string | NO | Represents the email address of the customer associated with the mandate request. |
4. Updating UI Web-Embeds
The reset method in UI Web-Embeds enables the updating of data within their instances while ensuring intelligent and seamless management of these changes.
This method is designed to be implemented across all currently mounted components.
<script type="text/javascript">
components.reset({
accessToken: "<newAccesstoken>"
});
</script>
5. DOM Event Listeners
UI Web-Embeds fire DOM events to let you know of any user action taking place within each UI Web-Embed but refer to other areas of your platform, so you could act according to the user’s intent.
Create DOM event listeners
Place the following script tag element at the very bottom, below the closing </body>
tag of your HTML page.
components.on("paymentsEnable", (e) => {
console.log("paymentsEnable event", e.detail);
});
components.on("startOnboarding", (e) => {
console.log("startOnboarding event", e.detail);
});
components.on("completeOnboarding", (e) => {
console.log("payPortal event", e.detail);
});
components.on("payPortal", (e) => {
console.log("payPortal event", e.detail);
});
components.on("invoicePage", (e) => {
console.log("payPortal event", e.detail);
});
components.on("buyerPage", (e) => {
console.log("payPortal event", e.detail);
});
components.on("onboardingFinished", (e) => {
console.log("onboardingFinished event", e.detail);
});
DOM events will be received in the following format, e.detail
, and may include a payload:
invoicePage
{
invoiceId: "INV1234"
}
Handle DOM events
Event | Description | Action | UI Web-Embeds |
---|---|---|---|
startOnboarding | This event is triggered when a user expresses their intent to register with your UNIPaaS-powered embedded payments solution. This event is applicable only to new users who have not yet started registration. | Redirect the user to the Create New Vendor flow on your platform Learn how to create a vendor | Pay Portal, Notification, Invoice, Balance |
completeOnboarding | This event is triggered when a user expresses their intent to complete registration with your UNIPaaS-powered embedded payments solution. | Redirect the user to the UNIPaaS Embedded Onboarding Component / Hosted Onboarding link Learn more about vendor onboarding | Pay Portal, Notification, Invoice, Balance |
invoicePage Payload: {invoiceId: "{string}" | This event is triggered when a user expresses their intent to view a specific invoice. The event includes the invoice number as a payload. | Redirect a user to the relevant invoice page in your platform | Pay Portal, Notification |
buyerPage Payload: {buyerId: "{string}"} | This event is triggered when a user expresses their intent to view a specific customer's profile. The event includes the buyer ID as a payload. | Redirect a user to the relevant customer page in your platform | Pay Portal, Notification |
payPortal Payload: {queryParam?: "{string}"} | This event is triggered when a user expresses their intent to view the Pay Portal (embedded component) or a specific tab of it. The event may include a query parameter as a payload. Example: {queryParam?: "page=payments"} | Redirect the user to the UNIPaaS embedded Pay Portal in your platform. If a query parameter is included, add it to the page URL's query string | Notification, Balance |
enablePayments | This event is triggered when a user expresses their intent to enable the UNIPaaS-powered embedded payments solution in your platform when it is disabled | Redirect the user to the settings page in your platform, where they can enable the payment solution and set it as a default payment option | Invoice |
onboardingFinished Payload: {status: "{string}" | This event is triggered when a user finishes filling out the onboarding fields and presses "Finish" after submitting the onboarding form. The event includes an onboarding status as a payload. Example: {status: "IN_REVIEW"} Learn more about onboarding statuses | Handle this event according to the user experience defined on your platform. For example, you can display a message to the user. If the onboarding form was opened as a modal window, you can close it. | Onboarding |
Updated about 1 year ago