Implement Direct Debit With UI Web-Embeds
General overview
If you are unfamiliar with Direct Debit and how it works in UNIPaaS, please refer to this article first.
In this section, you will be guided through the steps necessary to implement the Direct Debit payment method within your embedded payments solution, by leveraging the UNIPaaS UI Web-Embeds. Applying these will significantly reduce the integration effort required by your team to add Direct Debit to your offering.
The following UI Web-Embeds/services are required for Direct Debit to work:
- Invoice end point
- Invoice UI Web-Embed
- Pay Portal UI Web-Embed
- Notification UI Web-Embed OR notification/create webhooks
- Mandate Request UI Web-Embed(only for a mandate request flow outside of the Invoice UI Web-Embed)
Sequence diagram for a Direct Debit collection flow:
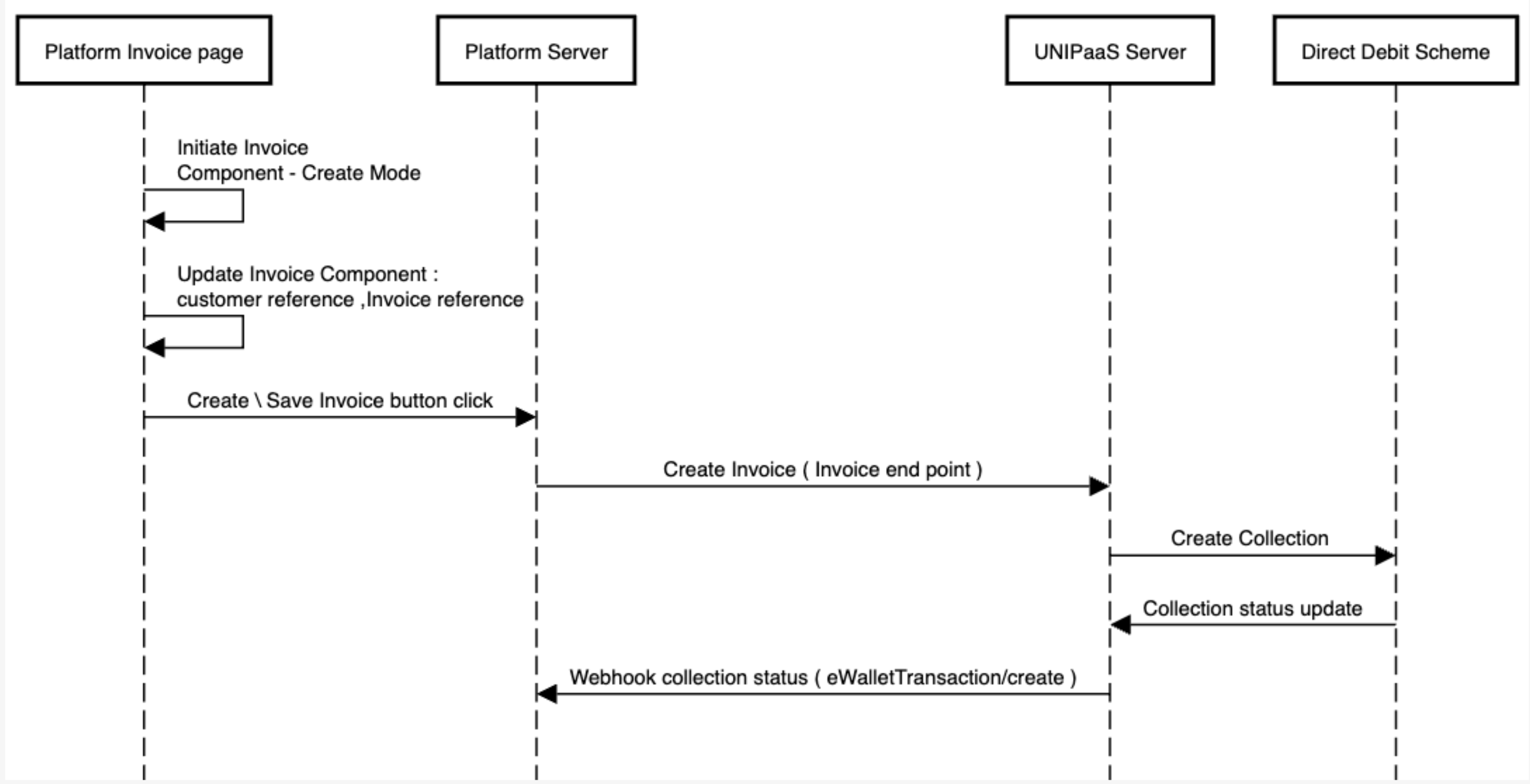
How to implement
Invoice endpoint
- In order to trigger Direct Debit collections (both one-off and recurring), it is required to use the invoice endpoint. This provides us with the relevant information for a Direct Debit collection.
- Learn about this service and how to implement it here.
- Handling recurring invoices - see here.
- UNIPaaS will support changes made to invoices that are due to be collected via Direct Debit. We currently support changes in invoice amount or due date. You will need to inform us of these changes via the update invoice method.
- Invoice endpoint attributes:
Name | Is Required | Type | Description |
---|---|---|---|
currency | Yes | ISO 4217 | The currency of the invoice |
totalAmount | Yes | Number | The total invoice amount |
totalPaid | No | Number | The total amount paid so far on this invoice. If not provided, will be set to 0 by default. |
reference | Yes | String | The invoice number on your system. |
batchId | No | String | The ID of the batch that this invoice was included in. |
vatAmount | No | Number | The total VAT amount for the invoice (can be 0). If not provided, will be set to 0 by default. |
publicUrl | No | String | A public URL to the invoice PDF version. |
dueDate | Yes | Date | The invoice due date. |
lineItems | Yes | Array | Line item object - see table below. |
Customer | Yes | Object | Customer object - see table below. |
paymentStatus | Yes | Enum | Current invoice payment status: Unpaid = unpaid Paid = paid Partially Paid = partially_paid Refunded = refunded PartiallyRefunded = partially_refunded |
paymentMethods | No | Array, Enum | States which payment methods will be available for this specific invoice. Note: If Direct Debit is selected, the invoice cannot be paid with UNIPaaS in any other method. Available Payment Methods: creaditCard bankTransfer directDebit offlineBankTransfer paypal Important - when the Invoice UI Web-Embed is not implemented (e.g., in the recurring payment flow), it is critical to send this parameter with directDebit for every recurring payment if Direct Debit is to be used to collect the payment.*Based on platform configuration |
invoiceObject | No | Object | The invoice object in its original form as created on your platform. |
isRecurring | Yes | Boolean | Indicates if this invoice is a recurring one. |
subscriptionId | No | String | The recurring invoice subscription identifier. |
lineItem
attributes:
Name | Is Required | Type | Description |
---|---|---|---|
description | Yes | String | A description for a specific line item |
amount | Yes | Number | A price of a unit for a specific line item |
quantity | Yes | Number | The quantity of a unit for a specific line item |
customer
attributes:
Name | Is Required | Type | Description |
---|---|---|---|
reference | Yes | String | Platform customer identifier. |
name | Yes | String | The customer full name. |
id | No | String | UNIPaaS customer identifier. |
email | No | String | The customer's email address. |
Invoice
- Learn about this UI Web-Embed here and learn how to install and implement it here.
- Add invoice mode (
create
/edit
/view
), as described in the implementation guide. Mandatory. - Add invoice reference in all invoice modes that exist in your platform (
create
,edit
,view
). Mandatory. - Add customer reference. This lets UNIPaaS know if the customer has a Direct Debit mandate set up with the vendor. Mandatory.
Pay Portal
- Learn about this UI Web-Embed here and learn how to install and implement it here.
- There are no Direct Debit considerations for this implementation.
Mandate Request
- Learn about this UI Web-Embed here and learn how to install and implement it here.
- Ensuring that Direct Debit is enabled for the vendor is highly important before presenting them with the Mandate Request UI Web-Embed. This verification can be achieved through the GET/vendors/{vendorId}/payment-options/available endpoint.
Notification
- Learn about this UI Web-Embed here and learn how to install and implement it here.
- There are no Direct Debit considerations for this implementation.
Note
If you don't implement the Notification UI Web-Embed, you will need to implement the notification/create webhook and implement it in the flow of your choice. See more on the notification/create service here.
Tip
We strongly recommend implementing a mandate set up flow from your vendors' customer list. See API implementation guide for more details.
Example of UI Web-Embeds implementation:
<!DOCTYPE html>
<html lang="en">
<head>
<script type="application/javascript" src="https://cdn.unipaas.com/embedded-ui.js"></script>
<meta charset="UTF-8">
<title>Direct Debit</title>
</head>
<body>
<h1>Invoice:</h1>
<div id="invoice"></div>
<h1>Request Mandate:</h1>
<div id="request_mandate"></div>
<h1>Pay Portal:</h1>
<div id="pay_portal"></div>
<h1>Notification:</h1>
<div id="notification"></div>
<script type="text/javascript">
async function getTokenToken() {
const url = "https://sandbox.unipaas.com/platform/authorize";
const secretKey = "gc2haOpujHMbKjAWk3UUWw==";
const vendorId = "64f8744b29095f715494b228";
const scopes = ["portal_read","portal_write","onboarding_write","invoice_read", "invoice_write"]
const options = {
method: "POST",
headers: {
"Accept": "application/json",
"Authorization": `Bearer ${secretKey}`,
"Content-Type": "application/json"
},
body: JSON.stringify({
scopes,
vendorId
})
};
try {
const response = await fetch(url, options);
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json();
return data.accessToken; // Assuming the access token is in the response
} catch (error) {
console.error('There was a problem with the fetch operation:', error);
return null;
}
}
async function initializeUniPAAS() {
const accessToken = await getTokenToken();
if (accessToken) {
const config_general = {
paymentsEnabled: true,
theme: {
colors: {
primaryColor: "#2F80ED",
secondaryColor: "#687B97",
accentTextColor: "#2F80ED",
primaryButtonColor: "#2F80ED"
},
fontFamily: "inherit",
boxShadow: "0px 3px 15px rgba(27, 79, 162, 0.11)"
}
}
const components = unipaas.components(accessToken, config_general);
const config_invoice = {
mode: 'create',
customer: {
reference: '1234xxx',
name: 'John Doe',
email: '[email protected]',
},
invoice: {
reference: 'INV-1234xxxx',
}
};
const invoice = components.create("invoice", config_invoice);
invoice.mount("#invoice");
const config_mandate = {
customer: {
reference: '1234xxx',
name: 'John Doe',
email: '[email protected]',
}
};
const request_mandate = components.create("requestMandate", config_mandate);
request_mandate.mount("#request_mandate");
const payPortal = components.create("payPortal");
payPortal.mount("#pay_portal");
const config_notif = {
icon: {
url: 'https://cdn.unipaas.com/img/bell-icon.svg',
size: 30
}
};
const notification = components.create("notification", config_notif);
notification.mount("#notification");
}
}
initializeUniPAAS();
</script>
</body>
</html>
Updated over 1 year ago