Buyer UI: Subscription Checkout Component
The Subscription Checkout Component will manage the UI logic, including displaying available payment methods, handling plan details, and determining the flow based on whether the user is new or returning.
Implementation Steps
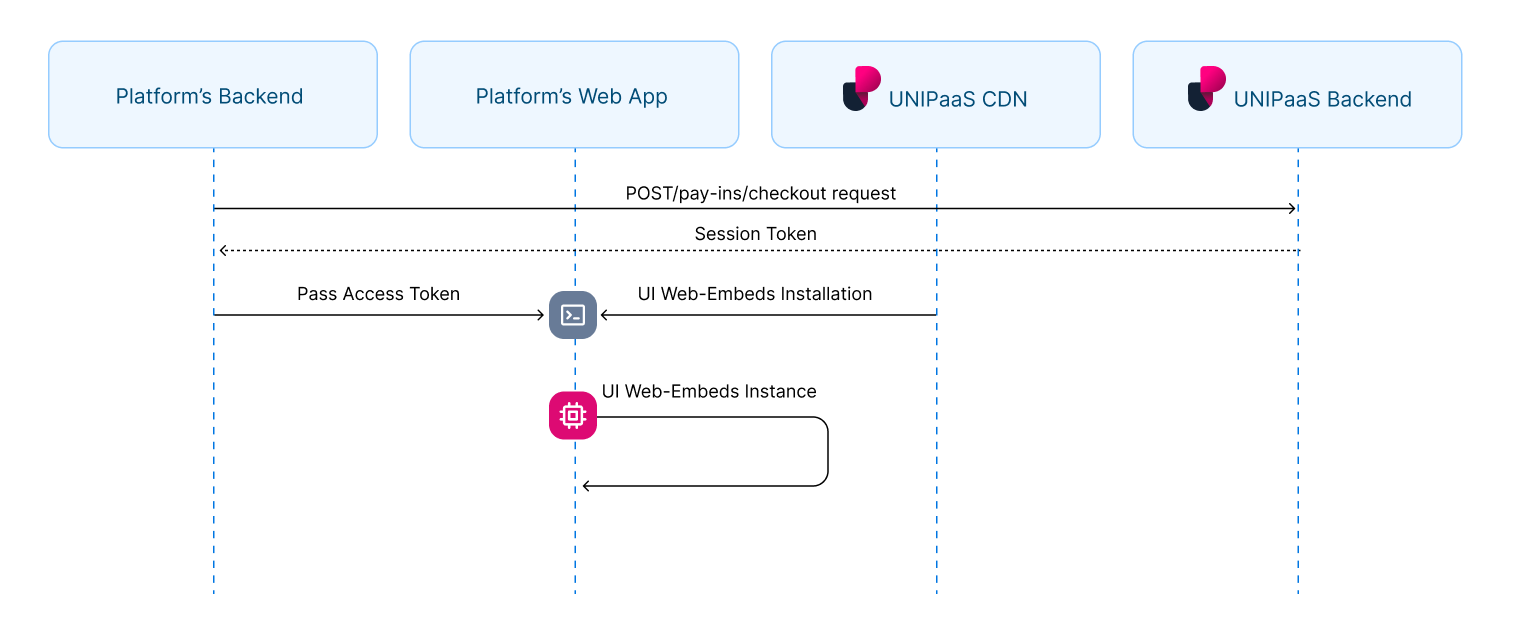
1. Authorization
In order to load the Subscription Checkout Component in your platform, an authorized API call is needed in your backend.
Generate an session token
To obtain an session token, you need first create a checkout using POST/pay-ins/checkout endpoint.
Learn more about payment creation
Below is an example of the JSON payload for creating a checkout:
{
"country": "GB",
"email": "[email protected]",
"paymentMethods": ["creditCard"],
"reference": "Payment123",
"description": "Payment for service",
"consumer": {
"name": "consumer.name",
"reference": "CON-REF-123"
},
"plans": [
"673e057913866a9ece78c2b1"
]
}
In response to this API call, you will receive a session token along with other parameters:
"sessionToken": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJtZXJjaGFudElkIjoiNjEwNjZkYjA2MzBmNGUzNTlkYzA4NGU5IiwibWVyY2hhbnROYW1lIjoiQWxpY2UiLCJhbW91bnQiOjEwMCwiY3VycmVuY3kiOiJHQlAiLCJlbWFpbCI6InVzZXJAZXhhbXBsZS5jb20iLCJjb3VudHJ5IjoiR0IiLCJzZWxsZXJJZCI6IjY2NWVkMjEyZmNkZjBjZmVjM2M4N2UwMiIsInZlbmRvcklkIjoiNjY1ZWQyMTJmY2RmMGNmZWMzYzg3ZTAyIiwic2NvcGVzIjpbIndlYnNka19hY2Nlc3MiLCJkaXJlY3RfZGViaXRfcmVhZCJdLCJpc1JlY3VycmluZyI6ZmFsc2UsInBheW1lbnRMaW5rSWQiOiJMa1FUMTJnTC1VIiwiaWF0IjoxNzE4NjA0ODk3LCJleHAiOjE3MTg2MDY2OTd9.tfW5IUKOdes4Lb0g5laFCyFZzySzfwe0gHo6Ohi2pqI"
This session token is necessary for loading the Subscription Checkout Component.
2. Installation
Script tag
Start with placing the following script tag element inside of the <head>
of your HTML page:
<script type="application/javascript" src="https://cdn.unipaas.com/embedded-components.js"></script>
This script tag loads the JavaScript code that provides the functionality for implementing UNIPaaS UI Web-Embeds on a webpage. When the script is loaded and executed, it will create an object in the memory that contains methods for instantiating and interacting with the UI Web-Embeds.
General configuration
Place the following script tag element below the closing <body>
tag of your HTML page.
This script is used to initialise and configure UNIPaaS UI Web-Embeds on a web page.
<script type="text/javascript">
const config_general = {
theme: {
type: 'dark',
variables: {
primaryBackgroundColor: '#121A2D',
secondaryBackgroundColor: '#18233B',
primaryTextColor: '#D8E2FB',
secondaryTextColor: '#7A92B5',
primaryButtonColor: '#2F80ED',
primaryButtonLabelColor: '#FFFFFF',
secondaryButtonColor: '#687B97',
buttonBorderRadius: '3px',
primaryInputBackgroundColor: '#18233B',
primaryInputBorderColor: '#3C517B',
primaryInputLabelColor: '#D8E2FB',
inputBorderRadius: '4px',
digitalWalletButtonMode: 'white',
},
},
}
const components = unipaas.buyerComponents("<accessToken>", config_general);
</script>
Theme
The theme configuration allows you to customise the visual design of UNIPaaS UI Web-Embeds to match your product's branding. The theme comes with a set of pre-defined variables that are automatically applied based on the selected theme type, such as "dark" or "default". However, if you need to modify specific aspects of the theme, you can easily adjust these variables to suit your requirements. The variables can be used to override the aspects of the theme.
Below is a table that explains each variable available for customization:
Name | Type | Description |
---|---|---|
theme.type | string | Specifies the theme type, such as "dark" or "default". |
primaryBackgroundColor | string | Defines the primary background color for the UI Web-Embeds. |
secondaryBackgroundColor | string | Sets the secondary background color for the UI Web-Embeds. |
primaryTextColor | string | Determines the color for primary text elements, including the checkout amount, vendor name, and payment method name. |
secondaryTextColor | string | Specifies the color for secondary text elements, including the reference number and save card for future payments. |
primaryButtonColor | string | Defines the color of the Pay button. |
primaryButtonLabelColor | string | Sets the label color on the Pay button. |
secondaryButtonColor | string | Determines the color of the Chevron for selecting the checkout language. |
buttonBorderRadius | string | Specifies the border radius of the payment button, influencing its roundness. |
primaryInputBackgroundColor | string | Defines the background color for input fields. |
primaryInputBorderColor | string | Specifies the border color for input fields. |
primaryInputLabelColor | string | Sets the label color for input fields. |
inputBorderRadius | string | Specifies the border radius for input fields, affecting their roundness. |
digitalWalletButtonMode | string | Determines the display mode for digital wallet buttons, such as "white" or "black". |
3. Implement Subscription Checkout Component on your webpage
The Embedded Subscription Checkout UI Web-Embed enables platforms to seamlessly integrate a subscription checkout flow directly into their website. This white-labeled component is fully customisable, allowing you to offer subscription plans with personalised details and descriptions, all within your platform's branding.
By embedding this component, you can enhance user experience streamline the subscription process, and accelerate your platform's recurring revenue offerings.
Allocate the minimal space for the Subscription Checkout Page Component as follows:
Width - 360 pixels
Create a container
Place the following
<div id="recurring"></div>
Create and mount an instance below the container
Create an instance of it and mount it to the container DOM node in your page. This should be done after the previous div has finished loading.
const recurring= components.create("recurring");
recurring.mount("#recurring");
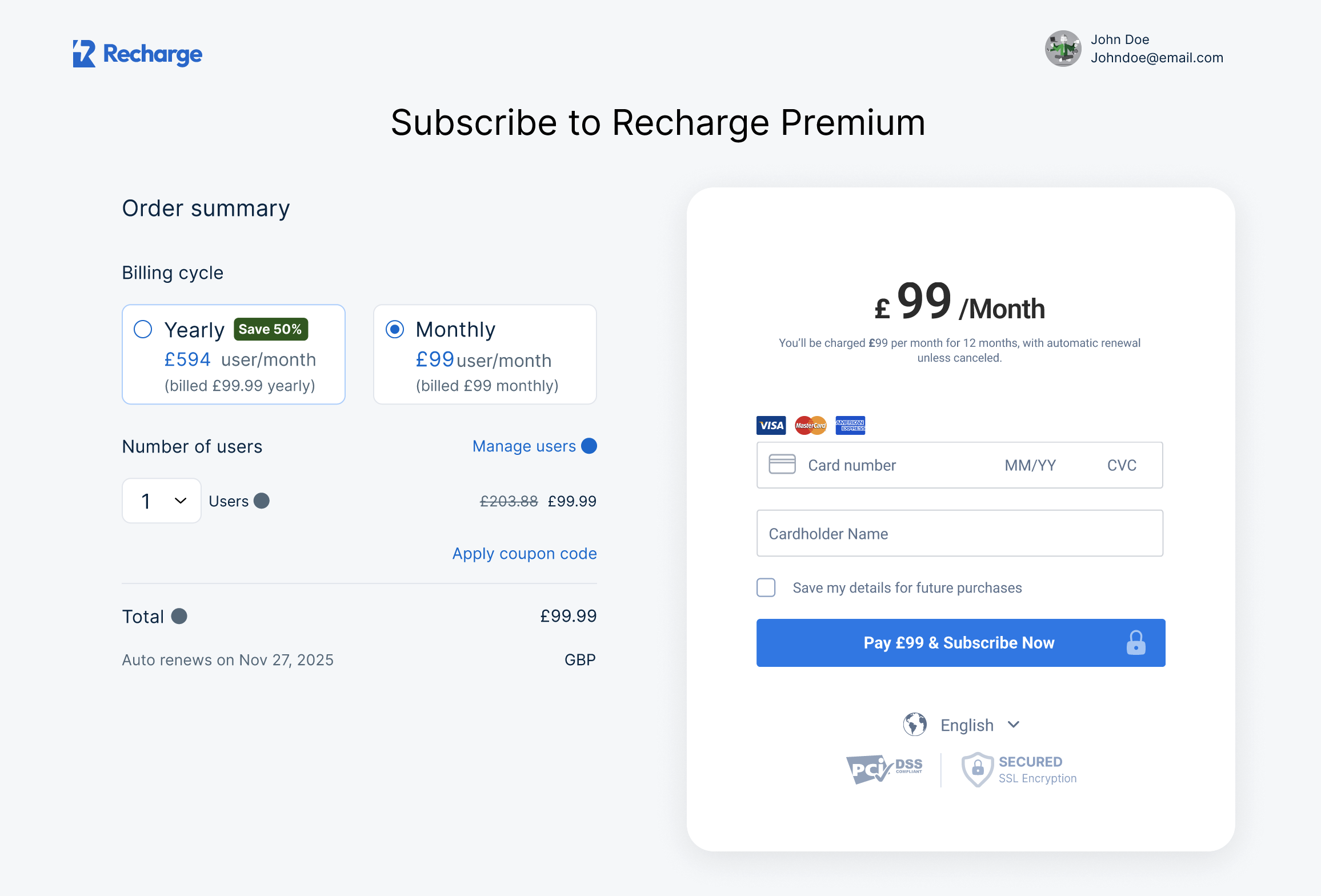
Example: The subscription checkout component is embedded in the website's checkout plan
4. DOM Event Listeners
The UI web-embeds generate DOM events to provide real-time updates on user activity and checkout status.
These events can be used to trigger custom actions and ensure a seamless user experience at platform's end.
components.on("paymentSuccess", (e) => {
console.log("paymentSuccess", e.detail);
});
components.on("paymentError", (e) => {
console.log("paymentError", e.detail);
});
components.on("paymentSubmission", (e) => {
console.log("paymentSubmission", e.detail);
});
components.on("paymentCancel", (e) => {
console.log("paymentCancel", e.detail);
});
Handle DOM events for Subscription Checkout Component
The Subscription Checkout Widget emits specific DOM events to provide real-time updates on the subscription process.
Create DOM event listeners
Place the following script tag element at the very bottom, below the closing </body>
tag of your HTML page.
components.on("subscriptionCreated", (e) => {
console.log("subscriptionCreated", e.detail);
// Handle successful subscription creation
// e.detail contains subscription details
});
components.on("subscriptionError", (e) => {
console.log("subscriptionError", e.detail);
// Handle subscription error
// e.detail contains error information
});
Handle DOM events
Event | Description | Action |
---|---|---|
subscriptionCreated | This event is triggered when a subscription has been created, and the first payment has been processed successfully. | Display a confirmation message or redirect to a welcome page. |
subscriptionError | This event is triggered when a subscription attempt encounters an error. | Handle errors appropriately and inform the user. |
Updated 2 months ago